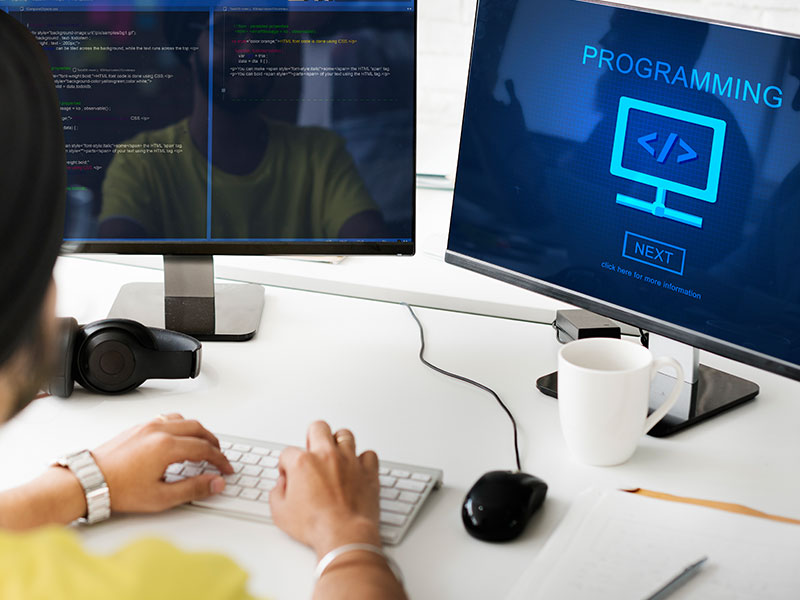
Object storage is a type of data storage architecture that manages data as distinct units, known as objects. Each object typically consists of data, metadata (descriptive information about the object), and a unique identifier. While deploying our code in the cloud, we must store our objects (images and videos) in the cloud storage and retrieve them from the object storage. In this tutorial, we will explain how to do it from a Spring Boot application.
Add the object storage dependency in the pom.xml file.
Change the version accordingly.

This is the controller for the image upload service.

This method is declared as a handler for the POST request. It accepts a single parameter of type MultipartFile named file. The @RequestBody annotation indicates that the file parameter is expected to be part of the request body. It returns a ResponseEntity <Response>, which is a Spring class representing the entire HTTP response, including the status code, headers, and body.
This method is in the service class to upload file to bucket in object storage.


You must provide your own access key and secret.
Explaination
- Input Parameters: The method takes two parameters – bucketName and fileName – representing the S3 bucket name and the file to be downloaded.
- Amazon S3 Client Configuration: It creates a ClientConfiguration object and sets the protocol to HTTPS. It initializes an AmazonS3 client using the provided AWS access key, secret key, and the configured client.
- Set S3 Endpoint: It sets the endpoint for the S3 client using the amazonS3Url.
- Check Object Existence: It uses s3.doesObjectExist to check if the specified object (file) exists in the S3 bucket.
- Download and Build Response: If the object exists, it proceeds to download the file using s3.getObject. It reads the object content in chunks and appends the bytes to the responseBytes array.The resulting byte array is returned as the response.
- Resource Clean up: It ensures proper closure of resources by calling objectContent.close() and s3Object.close() in a finally block.
Similarly, if we want to download file from object. You can follow the code below.


- Method Signature: public byte[] fileDownloadfromBucket(String bucketName, String fileName) throws IOException. It takes two parameters: bucketName (the name of the Amazon S3 bucket) and fileName (the name of the file within the bucket).
- AWS S3 Client Configuration: An instance of ClientConfiguration is created and configured to use HTTPS protocol. An instance of AmazonS3 is created using AWS credentials (accesskey and secret) and the configured client configuration.
- The endpoint for the Amazon S3 service is set using setEndpoint with the specified amazonS3Url.
- The file content is read into a byteArray using S3ObjectInputStream from the S3Object.The bytes are read in chunks, and the response byte array is dynamically resized and populated.
- Cleanup: The S3ObjectInputStream and S3Object are closed in a finally block to ensure proper cleanup.
For more information, please write to [email protected].